Introduction
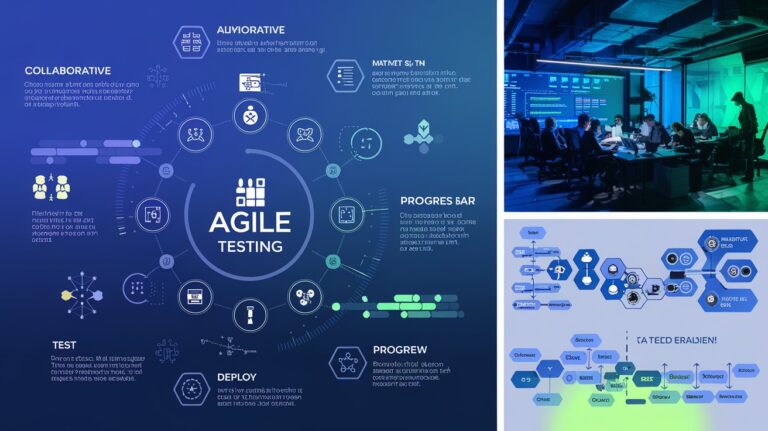
Selenium is a powerful tool for automating web applications, but ensuring efficiency, maintainability, and stability in test automation requires following best practices. Many testers face challenges such as flaky tests, slow execution, and high maintenance costs. By applying advanced best practices, teams can improve test reliability, execution speed, and overall framework efficiency.
This article covers the top advanced Selenium best practices to help you optimize your automation strategy.
1. Use the Page Object Model (POM) for Maintainability
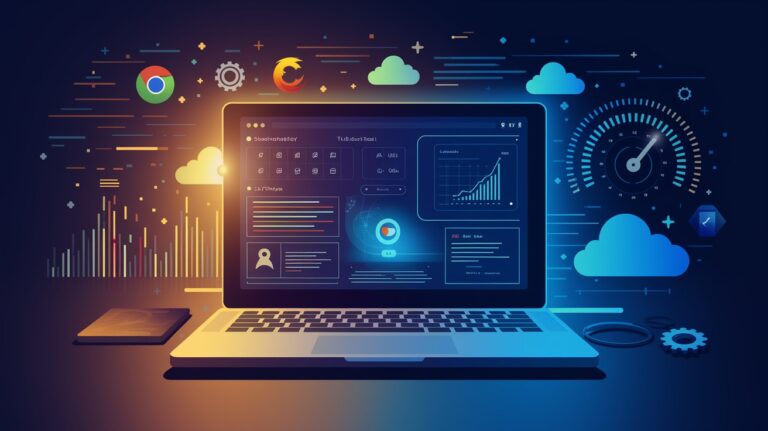
The Page Object Model (POM) is a widely used design pattern that improves test organization and maintainability by separating UI elements from test scripts.
Best Practices for POM
Create dedicated Page Object classes for different pages or modules.
Store locators and actions in separate classes to enhance reusability.
Use meaningful method names to improve code readability.
Implement a Base Page to handle common functionalities across pages.
Benefits:
Reduces test maintenance effort when the UI changes.
Enhances test readability and structure.
Improves code reusability and modularity.
2. Implement Explicit Waits Instead of Thread.sleep()
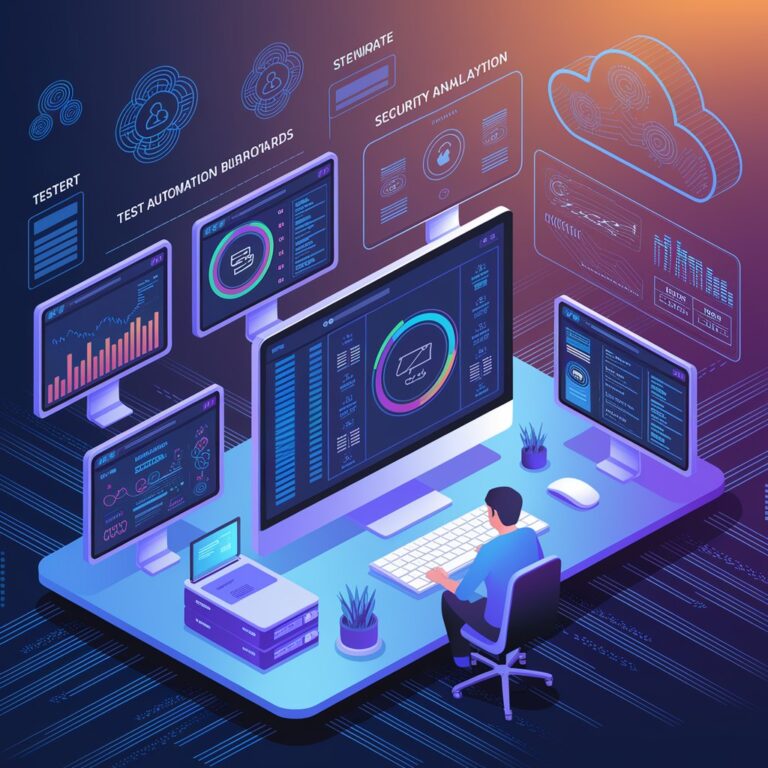
Using Thread.sleep()
can slow down test execution and introduce flakiness. Instead, Explicit Waits ensure synchronization by waiting for elements dynamically.
Best Practices for Using Waits
Use
WebDriverWait
with expected conditions instead of hardcoded delays.Implement Fluent Wait for polling elements dynamically.
Avoid using Implicit Waits in combination with Explicit Waits to prevent conflicts.
Example Expected Conditions:
visibilityOfElementLocated
– Waits for an element to become visible.elementToBeClickable
– Ensures the element is clickable.presenceOfElementLocated
– Waits for an element to appear in the DOM.
Benefits:
Improves test stability and execution speed.
Reduces test failures due to dynamic elements.
3. Use Headless Browsers for Faster Execution
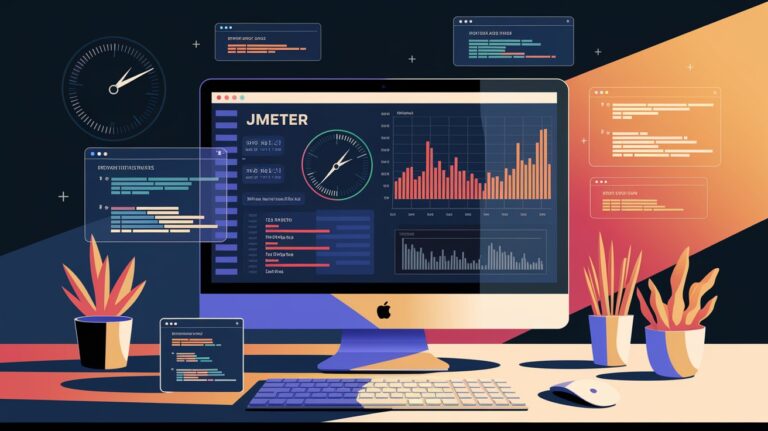
Headless testing allows browsers to execute tests without rendering the UI, improving execution speed.
Best Practices for Headless Testing
Use Chrome or Firefox headless mode for performance optimization.
Run headless tests in CI/CD pipelines to save execution time.
Combine headless mode with parallel execution to maximize efficiency.
Benefits:
Faster test execution.
Ideal for cloud environments and CI/CD integration.
4. Leverage Parallel Execution to Save Time
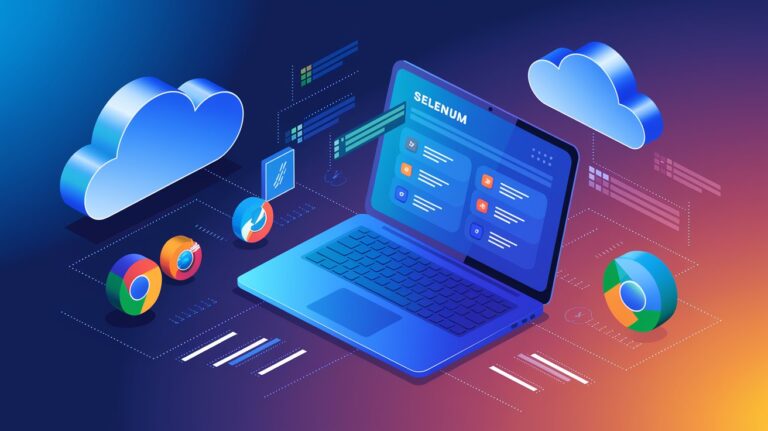
Parallel execution enables multiple tests to run simultaneously, significantly reducing test execution time.
Best Practices for Parallel Execution
Use TestNG, JUnit, or Selenium Grid for parallel execution.
Configure test frameworks to handle multiple test threads.
Utilize cloud-based Selenium Grids (e.g., BrowserStack, Sauce Labs) for scalability.
Benefits:
Saves execution time by running tests concurrently.
Enhances test coverage across browsers and devices.
5. Optimize Locator Strategies for Stability
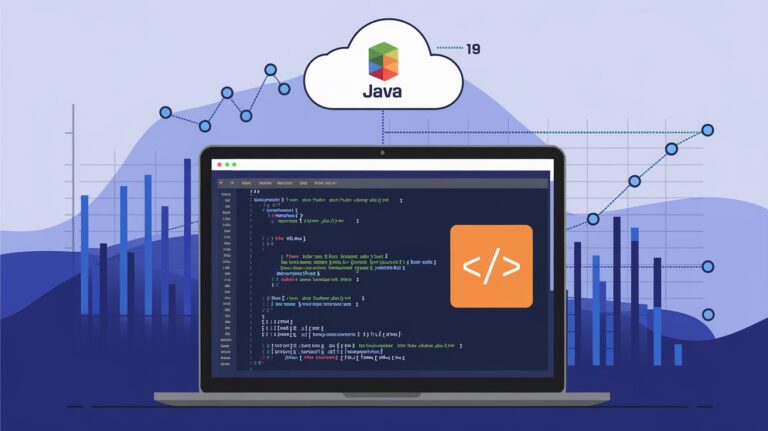
Choosing the right locator strategy improves test stability and reduces failures due to UI changes.
Best Practices for Locators
Prefer ID and Name locators for efficiency and uniqueness.
Use CSS Selectors over XPath for better performance.
Avoid using dynamic XPath expressions as they are prone to breakage.
Utilize custom attributes (
data-test-id
,aria-label
) when available.
Locator Preference Order:
ID – Fastest and most reliable.
Name – Reliable but may not always be unique.
CSS Selector – Flexible and efficient.
XPath – Use only when necessary.
Benefits:
Improves test execution speed.
Ensures higher test stability and robustness
6. Integrate Selenium with CI/CD Pipelines
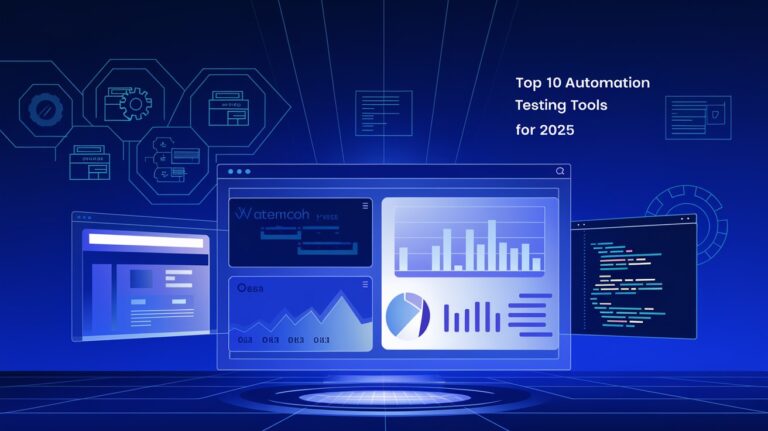
To enable continuous testing, integrate Selenium with CI/CD pipelines to automate tests on every code commit.
Best Practices for CI/CD Integration
Use Jenkins, GitHub Actions, GitLab CI/CD, or Azure DevOps for automation.
Generate test reports for detailed insights.
Run tests in Docker containers for consistency.
Configure notifications for test failures to detect issues early.
Benefits:
Faster feedback loops in development.
Automates regression testing efficiently.
7. Implement Data-Driven Testing for Scalability
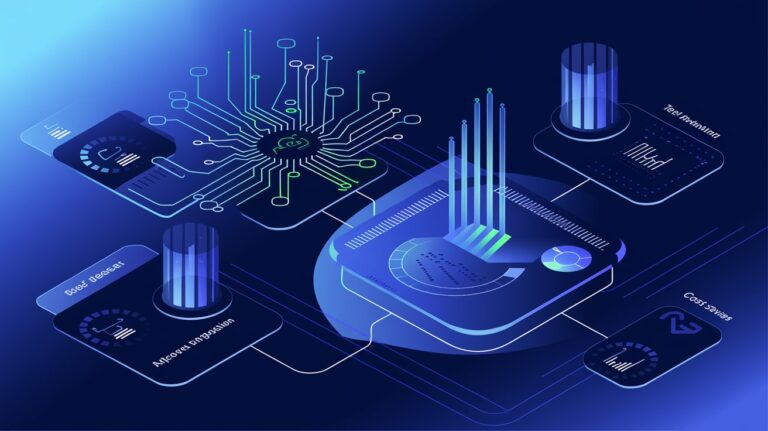
Instead of hardcoding test data, Data-Driven Testing (DDT) allows multiple test scenarios using external data sources.
Best Practices for Data-Driven Testing
Use external data sources such as Excel, JSON, CSV, or Databases.
Parameterize tests using JUnit, TestNG, or Apache POI.
Keep test logic and test data separate for better maintainability.
Benefits:
Reduces code duplication.
Supports multiple test cases with minimal modifications.
8. Capture Screenshots for Debugging
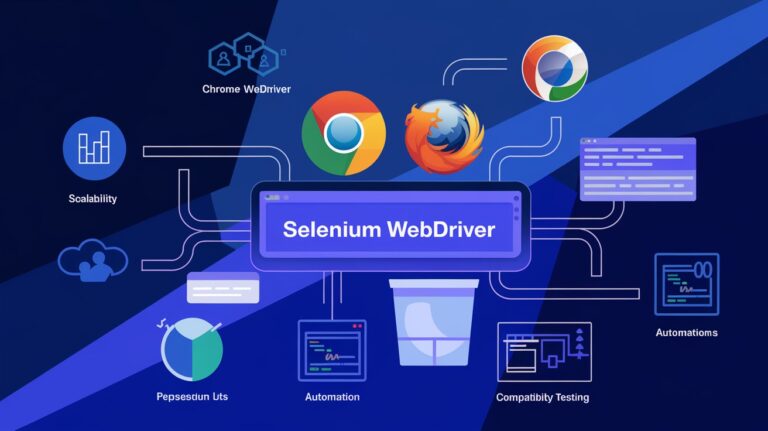
Capturing screenshots on test failures helps troubleshoot issues quickly.
Best Practices for Screenshot Capture
Take screenshots only on failures to save storage space.
Save images with meaningful filenames and timestamps.
Attach screenshots to test reports for better visibility.
Benefits:
Speeds up debugging and issue resolution.
Enhances the accuracy of test failure reports.
9. Use Logging and Reporting for Better Test Insights
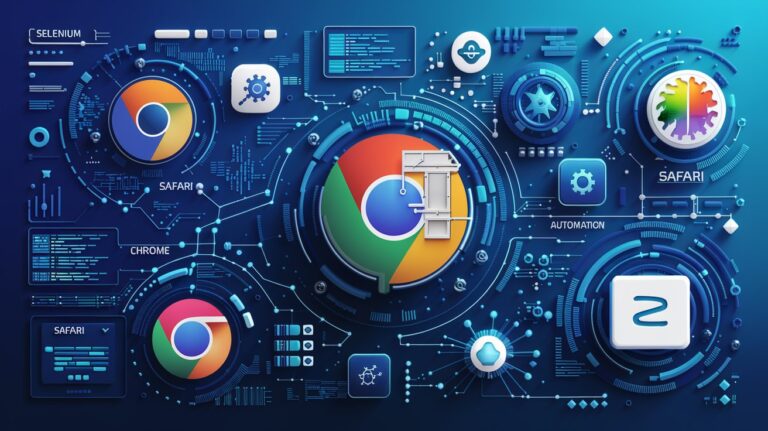
Logs and reports provide a detailed view of test execution, making it easier to analyze failures.
Best Practices for Logging and Reporting
Use Log4j or built-in logging frameworks for structured logs.
Integrate reporting tools like Allure, ExtentReports, or TestNG Reports.
Configure logging levels (INFO, DEBUG, ERROR) to capture necessary details.
Benefits:
Improves visibility into test execution.
Makes failure analysis easier and faster.
10. Secure Sensitive Data with Environment Variables
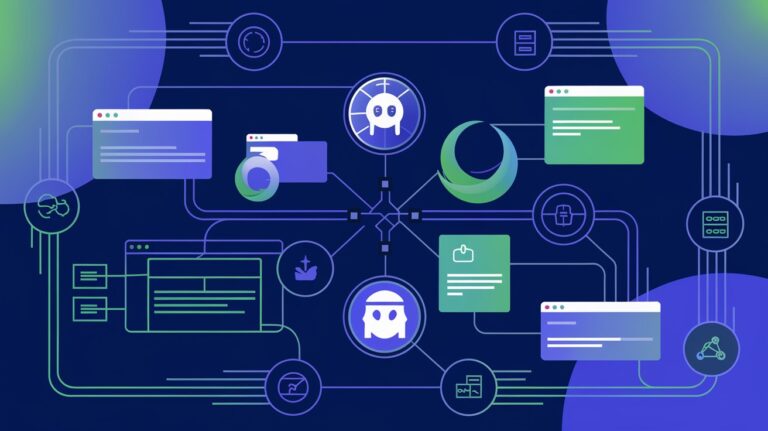
Avoid hardcoding credentials and sensitive data in test scripts to prevent security vulnerabilities.
Best Practices for Secure Data Handling
Store API keys, usernames, and passwords in environment variables.
Use configuration files (JSON, YAML, .properties) for sensitive information.
Implement encryption techniques to secure confidential data.
Benefits:
Prevents unauthorized access to sensitive data.
Ensures compliance with security best practices.
Conclusion
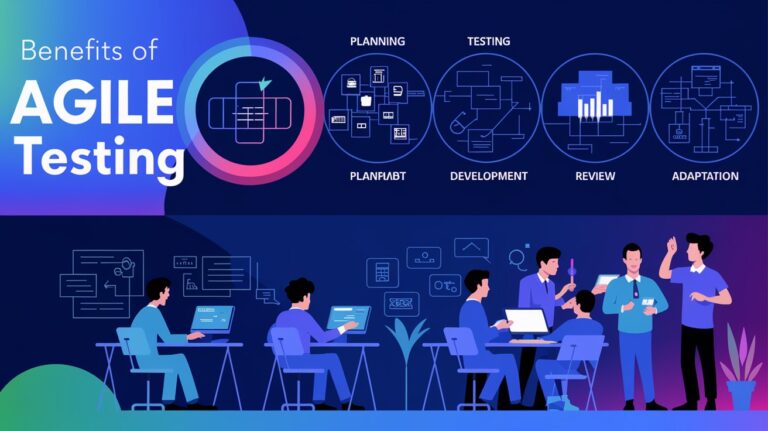
By following these advanced Selenium best practices, you can build efficient, scalable, and maintainable automation frameworks that improve test reliability and execution speed.
Key takeaways:
Implement Page Object Model (POM) for better maintainability.
Replace
Thread.sleep()
with Explicit Waits to improve test stability.Run tests in headless mode for faster execution.
Leverage parallel execution to optimize test time.
Optimize locator strategies to reduce flaky tests.
Integrate Selenium with CI/CD for automated regression testing.
Use data-driven testing to handle multiple scenarios effectively.
Capture screenshots, logs, and reports for better debugging.
Following these techniques will help you maximize the efficiency of Selenium automation while ensuring high-quality software testing.