Selenium has been a cornerstone of web automation testing for years, empowering QA engineers to ensure high-quality web applications. However, to truly harness its capabilities, you must follow best practices that ensure the efficiency, scalability, and maintainability of your tests. Here are 10 Selenium best practices every tester should know.
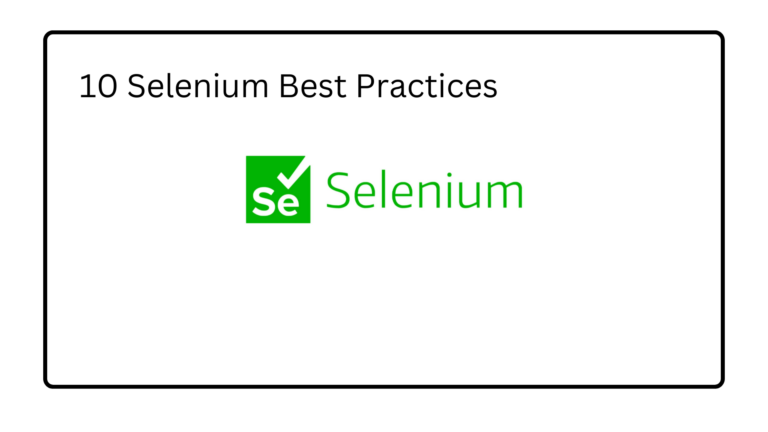
1. Use Explicit Waits Instead of Implicit Waits
Why: Implicit waits apply globally and can lead to unexpected delays if not configured properly. Explicit waits, on the other hand, are more targeted and efficient.
Example:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("submit")));
Best Practice: Use explicit waits to handle dynamic web elements and avoid hard-coded delays.
2. Keep Your Test Scripts Modular and Reusable
Why: Reusability reduces redundancy and simplifies maintenance.
How:
Break down test scripts into smaller, reusable components.
Use a Page Object Model (POM) to separate UI elements from test logic.
Example:
public class LoginPage { WebDriver driver; @FindBy(id = "username") WebElement usernameField; @FindBy(id = "password") WebElement passwordField; @FindBy(id = "login") WebElement loginButton; public LoginPage(WebDriver driver) { this.driver = driver; PageFactory.initElements(driver, this); } public void login(String username, String password) { usernameField.sendKeys(username); passwordField.sendKeys(password); loginButton.click(); } }
3. Use a Testing Framework
Why: A framework like TestNG or JUnit organizes test execution and reporting.
How:
Use annotations like
@Test
,@BeforeTest
, and@AfterTest
for better structure.Leverage built-in reporting tools for actionable insights.
Example:
@Test
public void testLogin() {
LoginPage loginPage = new LoginPage(driver);
loginPage.login("user", "password");
Assert.assertTrue(driver.getTitle().contains("Dashboard"));
}
4. Leverage Parallel Testing
Why: Running tests in parallel reduces execution time and ensures faster feedback.
How:
Configure parallel execution in TestNG or your preferred framework.
Use Selenium Grid or cloud services like BrowserStack.
Example (TestNG configuration):
<suite name="Suite" parallel="tests" thread-count="2"> <test name="Test1"> <classes> <class name="tests.TestClass1" /> </classes> </test> <test name="Test2"> <classes> <class name="tests.TestClass2" /> </classes> </test> </suite>
5. Avoid Hardcoding Test Data
Why: Hardcoding limits flexibility and makes tests brittle.
How:
Use external files (e.g., Excel, JSON, or properties files) to manage test data.
Example:
Properties properties = new Properties(); FileInputStream file = new FileInputStream("config.properties"); properties.load(file); String username = properties.getProperty("username"); String password = properties.getProperty("password");
6. Take Advantage of Headless Browsers
Why: Headless browsers like ChromeHeadless or PhantomJS run tests without opening a GUI, making them faster.
How:
Use headless mode for CI/CD pipelines to reduce resource consumption.
Example:
ChromeOptions options = new ChromeOptions(); options.addArguments("--headless"); WebDriver driver = new ChromeDriver(options);
7. Implement Proper Exception Handling
Why: Tests can fail due to unexpected issues; handling exceptions ensures stability.
How:
Use try-catch blocks to handle common exceptions like
NoSuchElementException
.Add meaningful error messages for debugging.
Example:
try { WebElement element = driver.findElement(By.id("submit")); element.click(); } catch (NoSuchElementException e) { System.out.println("Element not found: " + e.getMessage()); }
8. Use Assertions to Validate Results
Why: Assertions ensure the expected outcomes of tests.
How:
Use
assertTrue
,assertEquals
, and other assertion methods from frameworks like TestNG or JUnit.
Example:
String actualTitle = driver.getTitle();
String expectedTitle = "Dashboard";
Assert.assertEquals(actualTitle, expectedTitle, "Title does not match!");
9. Regularly Update WebDriver and Dependencies
Why: Keeping your WebDriver and libraries updated ensures compatibility with the latest browser versions and fixes known issues.
How:
Check for WebDriver updates regularly.
Use dependency management tools like Maven or Gradle.
Example (Maven dependency for Selenium):
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.8.1</version>
</dependency>
10. Leverage Browser Developer Tools
10. Leverage Browser Developer Tools
Why: DevTools provide insights into network activity, performance, and DOM structure, making debugging easier.
How:
Use Selenium’s DevTools API for advanced interactions.
Example:
DevTools devTools = ((ChromeDriver) driver).getDevTools(); devTools.createSession(); devTools.send(Network.enable(Optional.empty(), Optional.empty(), Optional.empty())); devTools.addListener(Network.requestWillBeSent(), request -> System.out.println("Request URL: " + request.getRequest().getUrl()));
Conclusion
By following these Selenium best practices, testers can write efficient, maintainable, and reliable test scripts. Whether you’re a beginner or an experienced tester, adhering to these guidelines will help you achieve better test coverage and faster delivery cycles.
What best practices do you follow when working with Selenium? Share your thoughts and experiences in the comments below!