Introduction
In today’s digital world, users access web applications from various browsers like Chrome, Firefox, Edge, and Safari. Ensuring a seamless experience across all browsers is essential. Cross-browser testing helps validate that web applications work consistently across different browsers, operating systems, and devices.
One of the most efficient ways to automate cross-browser testing is by using Selenium WebDriver. In this guide, we’ll explore how to set up and execute automated cross-browser testing with Selenium.
What is Cross-Browser Testing?
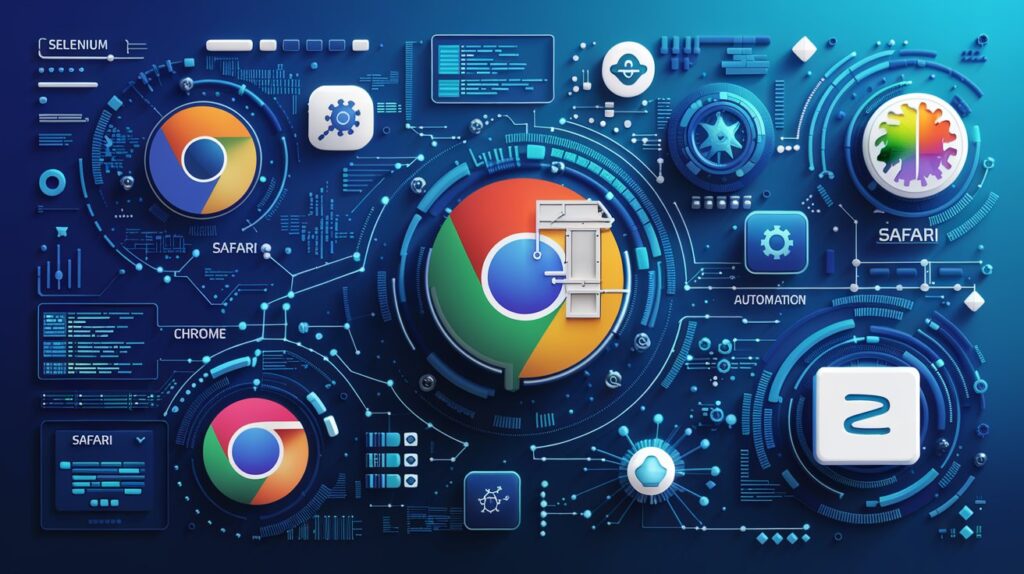
Cross-browser testing ensures that a web application:
✅ Renders correctly across different browsers and versions.
✅ Functions as expected across multiple platforms (Windows, macOS, Linux).
✅ Handles various screen sizes (responsive testing).
✅ Supports different rendering engines (Chromium, Gecko, WebKit).
Why is Cross-Browser Testing Important?
Different browsers interpret HTML, CSS, and JavaScript differently.
Users may experience UI glitches or broken functionality on certain browsers.
Some browsers support modern web features, while others require fallbacks.
Setting Up Selenium WebDriver for Cross-Browser Testing
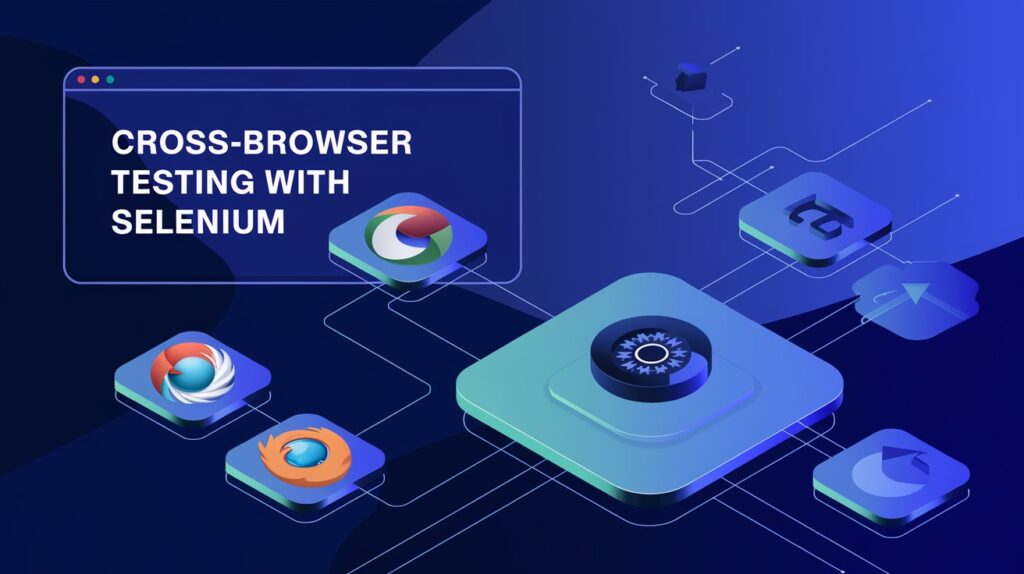
Step 1: Install Selenium WebDriver
Make sure you have the required dependencies installed. You can install Selenium for Java using Maven:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
Or install it for Python using pip:
pip install selenium
Step 2: Download Browser Drivers
Each browser requires a WebDriver to interact with Selenium:
Chrome → Chromedriver
Firefox → Geckodriver
Edge → Edge WebDriver
Safari → Comes pre-installed on macOS
Place the downloaded drivers in your system PATH or specify their location in your code.
Step 3: Write Cross-Browser Selenium Test Script
Here’s a Java example for running the same test on Chrome, Firefox, and Edge:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.edge.EdgeDriver;public class CrossBrowserTest {
public static void main(String[] args) {
String[] browsers = {“chrome”, “firefox”, “edge”};for (String browser : browsers) {
WebDriver driver = null;if (browser.equals(“chrome”)) {
System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver”);
driver = new ChromeDriver();
} else if (browser.equals(“firefox”)) {
System.setProperty(“webdriver.gecko.driver”, “path/to/geckodriver”);
driver = new FirefoxDriver();
} else if (browser.equals(“edge”)) {
System.setProperty(“webdriver.edge.driver”, “path/to/msedgedriver”);
driver = new EdgeDriver();
}driver.get(“https://yourwebsite.com”);
System.out.println(“Page Title on ” + browser + “: ” + driver.getTitle());driver.quit();
}
}
}
Step 4: Run Tests on Multiple Browsers
Save and run the script.
The test will execute on Chrome, Firefox, and Edge, opening and closing each browser.
Verify that elements load and function correctly in each browser.
Using Selenium Grid for Parallel Cross-Browser Testing
Running tests sequentially is time-consuming. To speed up cross-browser testing, use Selenium Grid, which allows parallel execution on multiple browsers and devices.
Steps to Set Up Selenium Grid
Download Selenium Server and start the Hub:
java -jar selenium-server-4.0.0.jar hub
2. Start Nodes for different browsers:
java -jar selenium-server-4.0.0.jar node –detect-drivers true
3. Modify your test script to connect to Selenium Grid:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.MalformedURLException;
import java.net.URL;public class SeleniumGridTest {
public static void main(String[] args) throws MalformedURLException {
String gridURL = “http://localhost:4444/wd/hub”;
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setBrowserName(“chrome”); // Change to “firefox” or “edge”WebDriver driver = new RemoteWebDriver(new URL(gridURL), capabilities);
driver.get(“https://yourwebsite.com”);System.out.println(“Page title is: ” + driver.getTitle());
driver.quit();
}
}
Best Practices for Effective Cross-Browser Testing
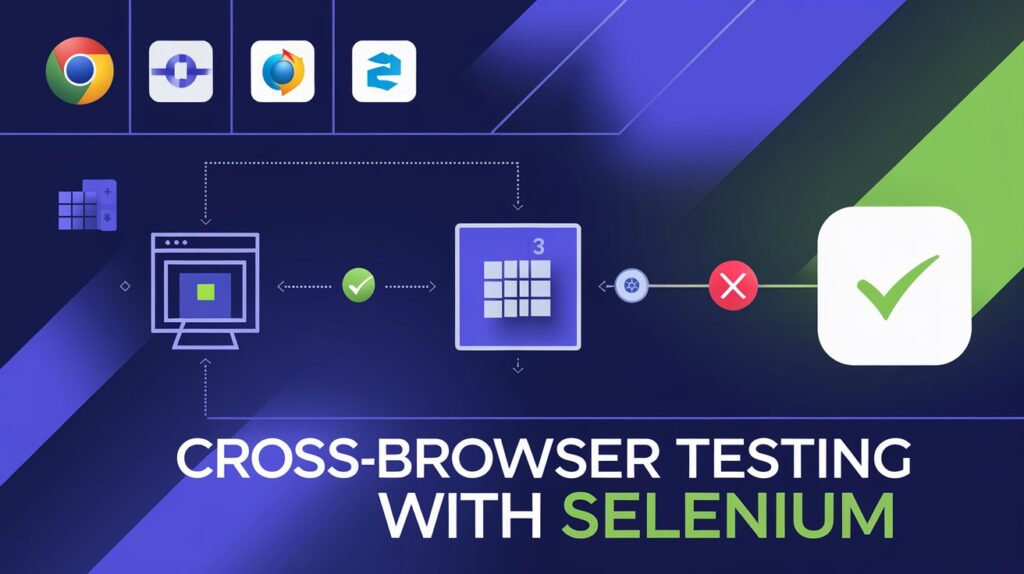
✅ Use BrowserStack or Sauce Labs for Cloud-Based Testing
Instead of setting up local infrastructure, you can use cloud-based services like:
These platforms provide access to multiple browsers and devices without complex setups.
✅ Test on Different Browser Versions
Older versions may lack support for modern CSS/JavaScript. Tools like LambdaTest can help test across different versions.
✅ Use Headless Browsers for Faster Execution
Running tests in headless mode (without opening a UI) speeds up execution:
ChromeOptions options = new ChromeOptions();
options.addArguments(“–headless”);
WebDriver driver = new ChromeDriver(options);
✅ Perform Responsive Testing
Test different screen sizes using:
driver.manage().window().setSize(new Dimension(375, 812)); // iPhone X resolution
Common Issues in Cross-Browser Testing & Solutions
Issue | Solution |
---|
Elements not displaying correctly | Use consistent CSS frameworks like Bootstrap. |
JavaScript execution differences | Use feature detection instead of browser detection. |
Tests failing due to dynamic elements | Use explicit waits (WebDriverWait). |
Inconsistent locators | Use CSS Selectors instead of fragile XPaths. |
Conclusion
Automating cross-browser testing with Selenium WebDriver helps ensure your web application delivers a consistent user experience across multiple browsers and devices. By leveraging Selenium Grid, headless testing, and cloud-based solutions, you can streamline the process and improve test coverage.