As software testing evolves, the demand for test automation continues to grow. For QA professionals, mastering programming skills—especially in Java—is no longer optional; it’s essential. Java’s versatility, ease of use, and wide range of libraries make it a top choice for automation testing frameworks. Whether you’re a beginner or looking to enhance your skill set, this article will guide you through the essential Java programming skills every QA tester needs to excel in automation testing
Why Java is Crucial for QA Automation
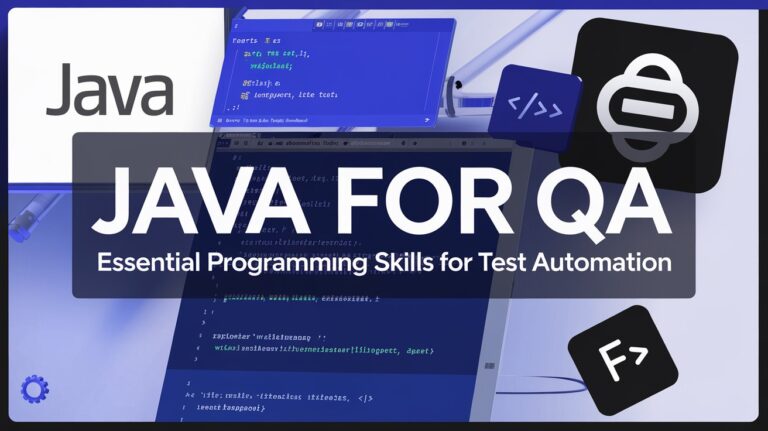
Versatility and Popularity
Java is platform-independent, making it suitable for cross-platform testing.
Its widespread adoption ensures abundant resources, libraries, and community support.
Integration with Testing Frameworks
Supports powerful automation frameworks like Selenium, TestNG, and JUnit.
Java-based frameworks streamline writing, executing, and managing test cases.
Career Advantages
Knowledge of Java enhances your marketability as a QA professional.
Employers prioritize QA testers skilled in Java due to its dominance in automation tools.
Essential Java Programming Skills for QA
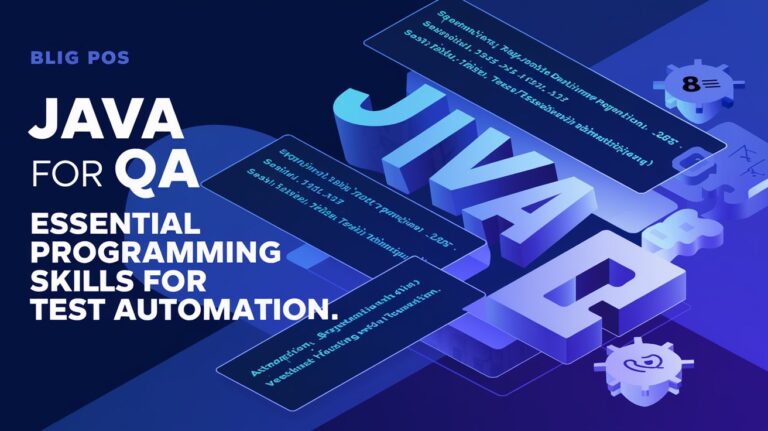
Core Java Concepts
Variables and Data Types:
Understand primitive and non-primitive data types.
Use variables effectively for test data manipulation.
Control Structures:
Master loops (for, while) and conditionals (if-else, switch) to handle test scenarios.
Object-Oriented Programming (OOP):
Grasp key concepts: inheritance, encapsulation, polymorphism, and abstraction.
Apply OOP principles to design reusable and maintainable test scripts.
Collections Framework:
Use Lists, Sets, and Maps to store and manipulate test data.
Exception Handling:
Implement try-catch blocks to handle runtime errors gracefully.
Advanced Java Skills for Test Automation
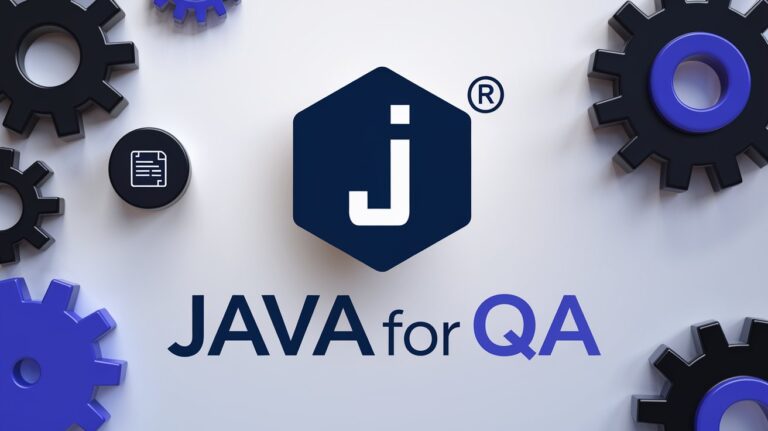
File Handling:
Read and write data from files for dynamic test input.
Use libraries like Apache POI for Excel file interactions.
Multithreading:
Execute parallel testing with multithreading concepts.
Java Streams and Lambda Expressions:
Process large datasets efficiently using streams.
Simplify code with lambda expressions for cleaner test logic.
Database Connectivity:
Use JDBC to validate test results against databases.
Getting Started with Java Frameworks for QA
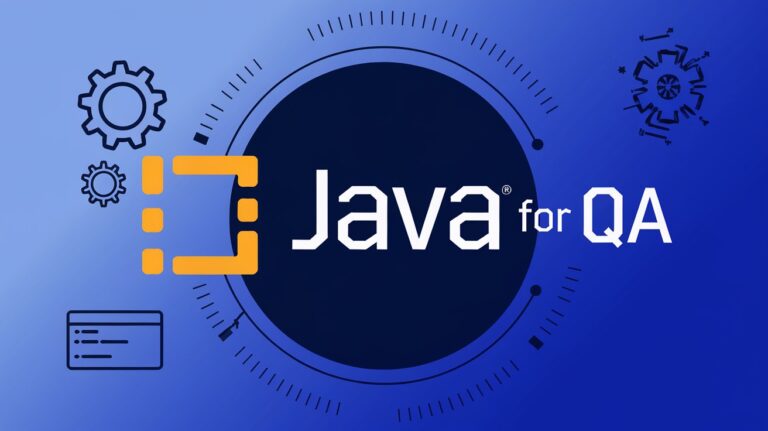
Selenium with Java
-
Automate web application testing using Selenium WebDriver.
-
Write scripts to handle browser interactions, form submissions, and UI validations.
TestNG for Test Management
-
Use TestNG annotations like @Test, @BeforeMethod, and @AfterMethod for organized test execution.
-
Generate detailed test reports for analysis.
JUnit for Unit Testing
-
Integrate JUnit with Selenium for robust test coverage.
Maven for Build Management
-
Manage dependencies and automate project builds.
Practical Example: Automating a Login Test Case
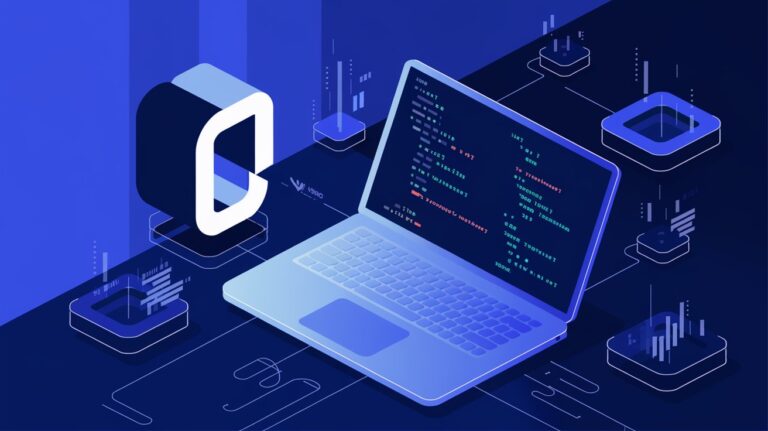
Step-by-Step Code:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;public class LoginTest {
public static void main(String[] args) {
// Set up WebDriver
System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver”);
WebDriver driver = new ChromeDriver();// Navigate to the login page
driver.get(“https://example.com/login”);// Perform login actions
driver.findElement(By.id(“username”)).sendKeys(“testuser”);
driver.findElement(By.id(“password”)).sendKeys(“password123”);
driver.findElement(By.id(“loginButton”)).click();// Validate login success
String expectedTitle = “Dashboard”;
if (driver.getTitle().equals(expectedTitle)) {
System.out.println(“Login test passed.”);
} else {
System.out.println(“Login test failed.”);
}// Close browser
driver.quit();
}
}
FAQs
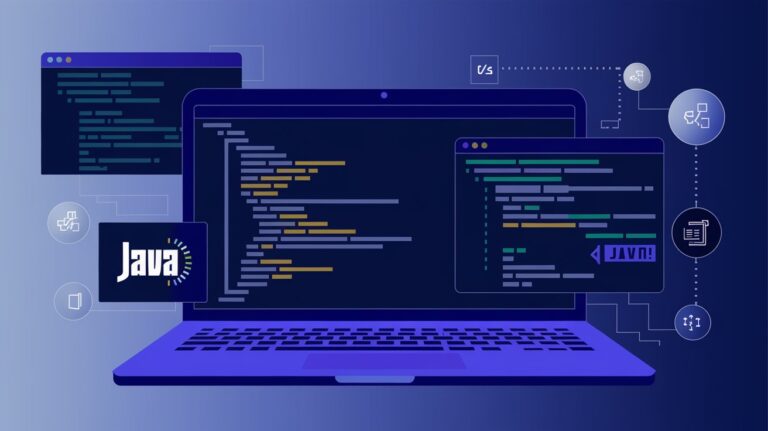
Q: Why should QA testers learn Java?
A: Java is the backbone of many automation frameworks like Selenium and TestNG, making it essential for QA professionals to write robust test scripts.
Q: Can I learn Java without prior programming experience?
A: Yes, Java is beginner-friendly, with extensive documentation and tutorials available for newcomers.
Q: What are the best Java libraries for QA automation?
A: Popular libraries include Apache POI (Excel handling), Selenium WebDriver, and TestNG for test management.
Conclusion
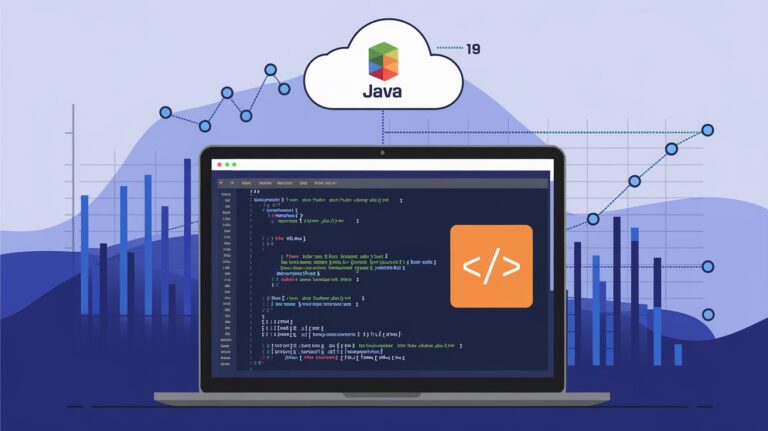
Mastering Java is a game-changer for QA professionals aspiring to excel in test automation. From core concepts to advanced skills, Java equips testers with the tools needed to write efficient and maintainable test scripts. By leveraging Java frameworks like Selenium and TestNG, you can streamline your testing processes and enhance your career prospects.
Ready to elevate your QA automation skills? Start learning Java today and unlock new opportunities in software testing!