Preparing for a Selenium-based QA role? Mastering advanced Selenium interview questions is crucial to demonstrating your expertise in automation testing. This guide provides real-world scenarios, tricky coding challenges, and valuable tips to help you ace your next interview.
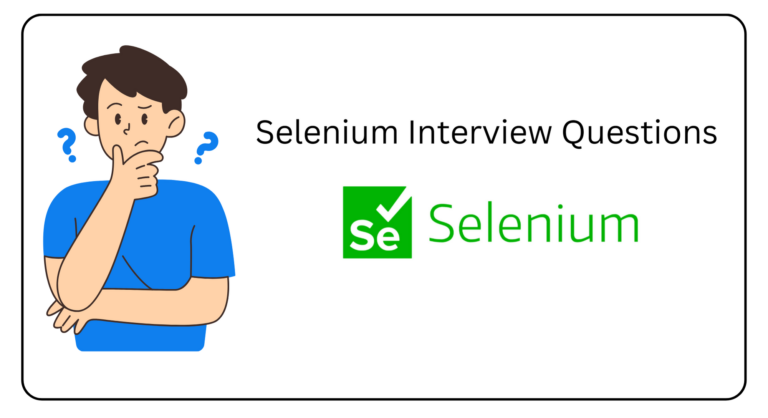
- Common Selenium Questions
- Advanced Questions with Real-world Examples
- Scenario-Based Questions
- Tricky and Edge Case Questions
- Coding Challenges in Selenium Interviews
- Tips for Answering Selenium Questions
- FAQs
Common Selenium Questions
1. What is Selenium WebDriver?
Answer:
Selenium WebDriver is a framework that allows testers to automate web application testing by controlling browsers programmatically. It provides APIs in multiple programming languages, including Java, Python, and C#.
2. What are the limitations of Selenium?
Answer:
Cannot test mobile applications directly (requires Appium for mobile testing).
Does not handle Windows-based popups.
Requires third-party tools for image-based testing.
3. What is the difference between findElement() and findElements()?
Answer:
findElement()
: Returns the first matching element on the web page. ThrowsNoSuchElementException
if no element is found.
findElements()
: Returns a list of all matching elements. If no elements are found, it returns an empty list.
4. How do you handle SSL certificate errors in Selenium?
Answer:
ChromeOptions options = new ChromeOptions();
options.setAcceptInsecureCerts(true);
WebDriver driver = new ChromeDriver(options);
Advanced Questions with Real-world Examples
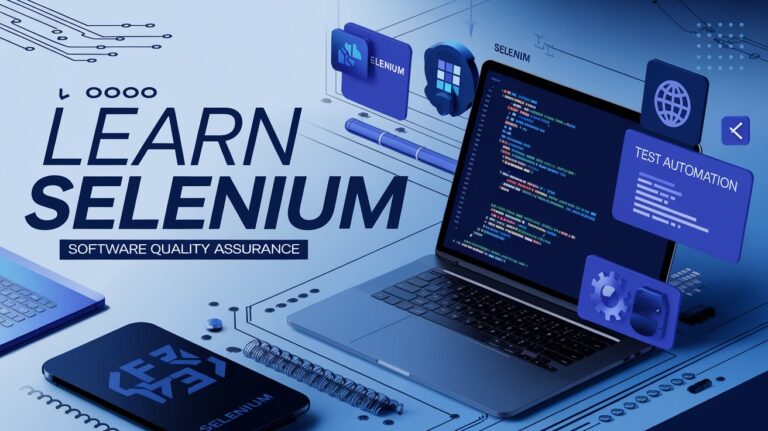
1. How do you handle dynamic web elements in Selenium?
Answer:
Use XPath functions like
contains()
,starts-with()
, ortext()
to locate dynamic elements. Example:
WebElement element = driver.findElement(By.xpath("//button[contains(text(), 'Submit')]");
2. How do you manage waits in Selenium?
Answer:
Implicit Wait: Applied globally for all elements.
Explicit Wait: Applied to specific elements.
Example:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("submit")));
3. How do you perform drag and drop in Selenium?
Answer:
Use the
Actions
class:Actions actions = new Actions(driver); WebElement source = driver.findElement(By.id("source")); WebElement target = driver.findElement(By.id("target")); actions.dragAndDrop(source, target).perform();
4. How do you capture screenshots in Selenium?
Answer:
Use the
TakesScreenshot
interface:TakesScreenshot screenshot = (TakesScreenshot) driver; File srcFile = screenshot.getScreenshotAs(OutputType.FILE); FileUtils.copyFile(srcFile, new File("screenshot.png"));
Scenario-Based Questions
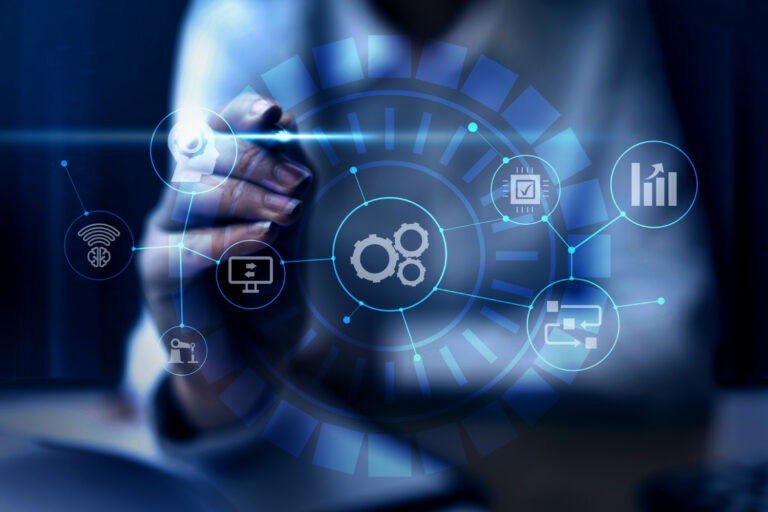
1. How would you handle a page where an element is not interactable?
Answer:
Scroll to the element using JavaScript:
JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript("arguments[0].scrollIntoView();", element);
Use
Actions
class to interact:Actions actions = new Actions(driver); actions.moveToElement(element).click().perform();
2. Describe how you would debug a failing test in a CI/CD pipeline.
Answer:
Check the logs for specific error messages.
Verify if the failure is due to environmental issues (e.g., browser version).
Run the test locally to isolate the issue.
3. How would you handle an alert popup in Selenium?
Answer:
Use the
Alert
interface:Alert alert = driver.switchTo().alert(); System.out.println(alert.getText()); alert.accept(); // To accept the alert
Tricky and Edge Case Questions
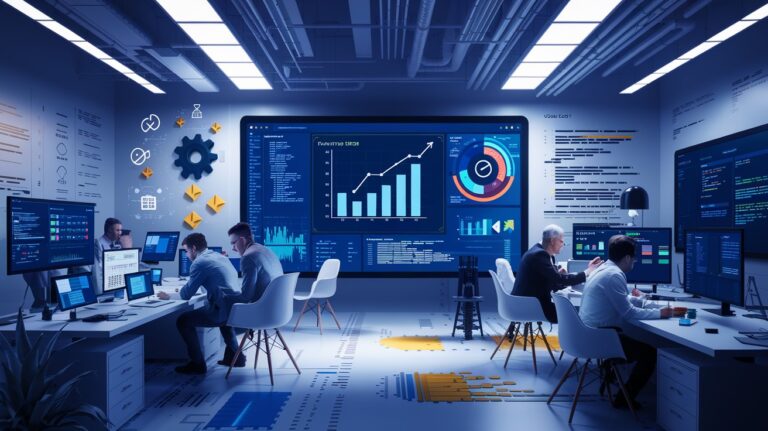
1. How do you verify tooltips in Selenium?
Answer:
Use the
getAttribute
method to fetch the tooltip text:WebElement tooltip = driver.findElement(By.id("tooltip")); String tooltipText = tooltip.getAttribute("title"); System.out.println("Tooltip text: " + tooltipText);
2. How do you handle multi-tab browsing in Selenium?
Answer:
Store all tab handles in a Set:
Set<String> handles = driver.getWindowHandles();
Switch to the desired tab:
for (String handle : handles) { driver.switchTo().window(handle); }
3. How do you verify the presence of a file after downloading it?
Answer:
Use Java to check the file system:
File file = new File("C:\\Downloads\\example.txt"); if (file.exists()) { System.out.println("File exists!"); } else { System.out.println("File does not exist."); }
Coding Challenges in Selenium Interviews
Write a Selenium script to upload a file.
Solution:
WebElement uploadButton = driver.findElement(By.id("file-upload")); uploadButton.sendKeys("C:\\path\\to\\file.txt");
Write a Selenium script to handle a dynamic dropdown.
Solution:
WebElement dropdown = driver.findElement(By.id("dropdown")); dropdown.click(); WebElement option = driver.findElement(By.xpath("//option[contains(text(), 'Option 2')]"); option.click();
Write a Selenium script to take a full-page screenshot.
Solution:
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE); FileUtils.copyFile(screenshot, new File("fullpage_screenshot.png"));
Tips for Answering Selenium Questions
-
Understand the Question:
-
Ask for clarification if needed.
-
Relate the question to real-world scenarios you’ve faced.
-
-
Be Concise:
-
Provide direct answers with examples or code snippets.
-
-
Highlight Experience:
-
Mention how you’ve used Selenium in previous projects (e.g., test frameworks, CI/CD integration).
-
FAQs
1. What are the must-know tools for Selenium testers?
Answer: Tools like TestNG, Maven, Jenkins, and Git are essential for Selenium testers.
2. How do I prepare for advanced Selenium interview questions?
Answer: Practice real-world scenarios, understand Selenium frameworks, and stay updated with new features.
3. What are some common Selenium interview mistakes?
Answer: Providing vague answers, not explaining code, and failing to demonstrate practical experience.
Advanced Selenium interview questions require a combination of technical knowledge, problem-solving skills, and practical experience. Use this guide to prepare thoroughly and stand out in your next QA interview.
For more resources, check out our Getting Started with Selenium and Automation Testing Tools guides!